The Boyer-Moore Algorithm: A Deep Dive into Efficient Pattern Matching
Pattern matching is a cornerstone of computer science, powering applications ranging from text editors and compilers to data retrieval and bioinformatics. Among the multitude of algorithms designed for this purpose, the Boyer-Moore algorithm stands out as one of the most efficient and elegant solutions. Developed in 1977 by Robert S. Boyer and J Strother Moore, this algorithm revolutionized string searching by introducing innovative heuristics that significantly reduce the number of character comparisons. This article delves into the intricacies of the Boyer-Moore algorithm, its components, and its practical applications.
Background and Importance
The Boyer-Moore algorithm is designed to locate all occurrences of a pattern string (referred to as the “pattern”) within a larger text string (referred to as the “text”). Its primary advantage lies in its ability to skip sections of the text, thereby avoiding redundant comparisons. While many pattern-matching algorithms scan the text from left to right, character by character, the Boyer-Moore algorithm employs a right-to-left scanning approach for the pattern, combined with two powerful heuristics: the bad character rule and the good suffix rule.
These heuristics make the algorithm particularly efficient for large texts and patterns, especially when the pattern is long or the alphabet is large. In the best-case scenarios, the algorithm achieves sub-linear time complexity, making it a preferred choice in scenarios where performance is critical.
Key Concepts and Terminology
Before diving into the mechanics of the Boyer-Moore algorithm, it is essential to understand some key terms and concepts:
- Pattern (P): The substring we aim to find within the larger text.
- Text (T): The string in which the pattern is searched.
- Shift: The distance by which the pattern is moved after a mismatch or a match.
- Alphabet (Σ): The set of possible characters in the text and pattern.
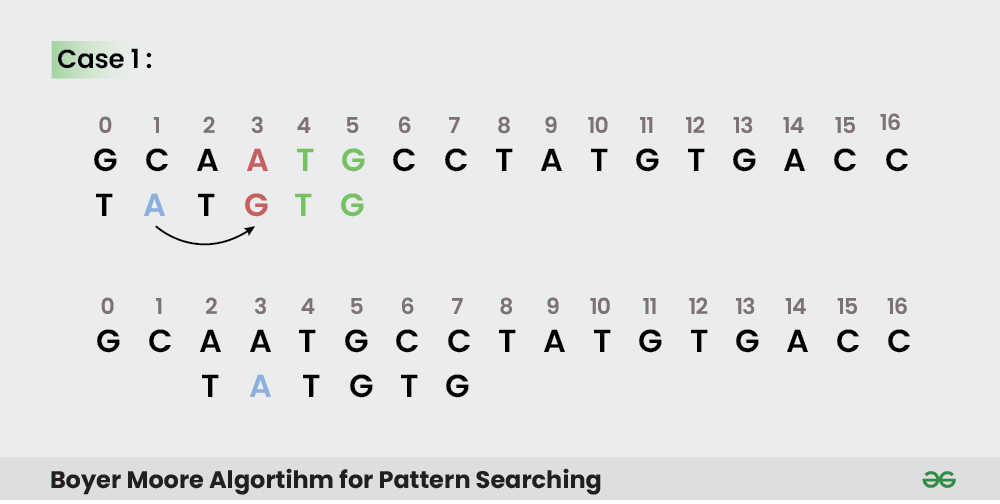
Core Components of the Algorithm
The Boyer-Moore algorithm leverages two primary heuristics:
1. The Bad Character Rule
The bad character rule is based on the observation that when a mismatch occurs during the pattern-text comparison, the mismatched character can be used to determine the shift. Specifically:
- Identify the position of the mismatched character in the text.
- Check if this character exists in the pattern.
- Shift the pattern so that the rightmost occurrence of this character in the pattern aligns with the mismatched character in the text.
- If the mismatched character does not exist in the pattern, shift the pattern completely past the mismatched character.
Example:
Let’s consider the text T = "GCTTCTGCTACCT"
and the pattern P = "CTAG"
:
- Start comparing the pattern from right to left with the text.
- If a mismatch occurs at the text character
T
and the character does not exist in the pattern, shift the pattern completely pastT
.
2. The Good Suffix Rule
The good suffix rule comes into play when a substring (suffix) of the pattern matches with a substring of the text, but a mismatch occurs at a preceding character. This rule determines how far the pattern can be shifted while still ensuring a valid match for the matched suffix.
- Identify the matched suffix in the pattern.
- Shift the pattern so that the next occurrence of this suffix in the pattern aligns with its position in the text.
- If the suffix does not occur elsewhere in the pattern, shift the pattern completely past the suffix.
Example:
Using the same text and pattern:
- Suppose the suffix
TA
of the pattern matches with the text. - If a mismatch occurs at the preceding character, shift the pattern so that the next occurrence of
TA
in the pattern aligns with the text.
Algorithm Steps
Here’s a step-by-step breakdown of the Boyer-Moore algorithm:
- Preprocessing:
- Compute the bad character table: For each character in the alphabet, store the index of its last occurrence in the pattern.
- Compute the good suffix table: For each possible suffix of the pattern, calculate the shift required to align the pattern with the text.
- Pattern Matching:
- Align the pattern with the beginning of the text.
- Compare characters of the pattern with the text from right to left.
- If a mismatch occurs, use the bad character rule and good suffix rule to determine the shift.
- If a match occurs, record the position and shift the pattern using the good suffix rule.
- Termination:
- Repeat the above steps until the pattern exceeds the length of the text.
Time Complexity Analysis
The efficiency of the Boyer-Moore algorithm stems from its ability to skip large portions of the text. Its time complexity can be analyzed as follows:
- Preprocessing Time:
- Computing the bad character table requires , where is the length of the pattern and is the size of the alphabet.
- Computing the good suffix table requires .
- Matching Time:
- In the best case, the algorithm achieves , where is the length of the text and is the length of the pattern. This occurs when the pattern’s shifts cover most of the text without redundant comparisons.
- In the worst case, the time complexity is , making it linear. This typically happens with specific patterns and texts that cause frequent mismatches.
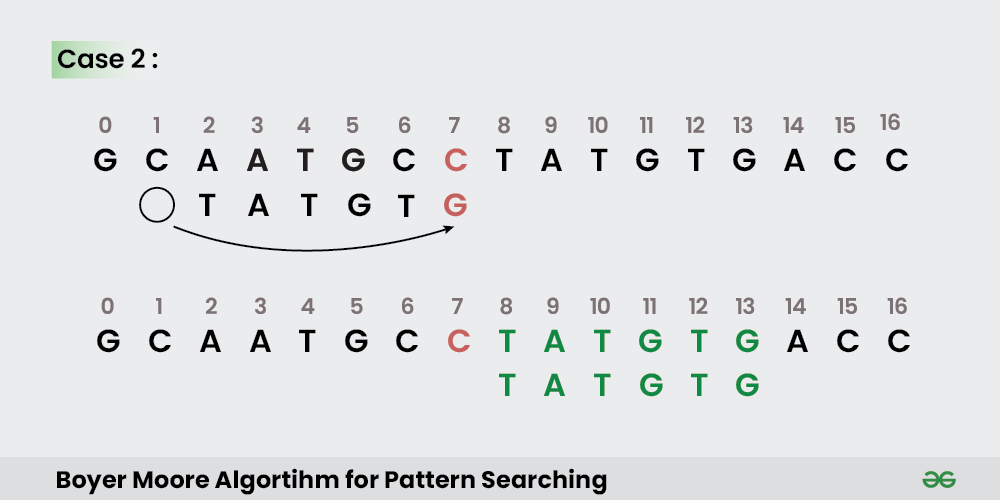
Practical Applications
The Boyer-Moore algorithm finds applications across various domains:
- Text Editors: Efficiently searching for words or phrases in documents.
- Compilers: Identifying patterns in source code during lexical analysis.
- Bioinformatics: Searching for DNA or protein sequences within large genomes.
- Plagiarism Detection: Finding copied text across documents.
- Cybersecurity: Detecting patterns of malicious activity in logs or network data.
Advantages and Limitations
Advantages:
- High efficiency for large texts and patterns.
- Sub-linear performance in best-case scenarios.
- Minimal overhead due to preprocessing.
Limitations:
- Performance may degrade for small patterns or when the alphabet size is small.
- Requires additional memory for preprocessing tables.
- Complex implementation compared to simpler algorithms like the Naïve or Knuth-Morris-Pratt algorithms.
Optimizations and Variants
Several optimizations and variants of the Boyer-Moore algorithm have been proposed to address its limitations and extend its applicability:
- Simplified Boyer-Moore: Focuses on the bad character rule alone, simplifying implementation.
- Boyer-Moore-Horspool Algorithm: A variant that uses only the bad character rule but optimizes it for better average-case performance.
- Tuned Boyer-Moore: Tailors the algorithm to specific hardware or data characteristics for improved performance.
Implementation in Code
def boyer_moore(text, pattern):
def preprocess_bad_character(pattern):
bad_char = {}
for i, char in enumerate(pattern):
bad_char[char] = i
return bad_char
def preprocess_good_suffix(pattern):
m = len(pattern)
good_suffix = [m] * m
last_prefix = m
for i in range(m - 1, -1, -1):
if pattern[i:] == pattern[:m - i]:
last_prefix = i + 1
good_suffix[m - i - 1] = last_prefix
return good_suffix
bad_char = preprocess_bad_character(pattern)
good_suffix = preprocess_good_suffix(pattern)
n, m = len(text), len(pattern)
i = 0
while i <= n - m:
j = m - 1
while j >= 0 and pattern[j] == text[i + j]:
j -= 1
if j < 0:
print(f"Pattern found at index {i}")
i += good_suffix[0]
else:
bad_char_shift = j - bad_char.get(text[i + j], -1)
good_suffix_shift = good_suffix[j] if j < m - 1 else 1
i += max(bad_char_shift, good_suffix_shift)
Conclusion
The Boyer-Moore algorithm exemplifies the power of combining innovative heuristics to achieve computational efficiency. Its enduring relevance in fields like text processing, bioinformatics, and cybersecurity underscores its significance. While it may not always be the ideal choice for every scenario, understanding its mechanics provides valuable insights into algorithm design and optimization. By mastering the Boyer-Moore algorithm, developers and researchers can harness its potential to tackle complex pattern-matching challenges with elegance and efficiency.
Questions and Answers
Q1: What is the primary purpose of the Boyer-Moore algorithm?
A: The Boyer-Moore algorithm is designed to efficiently locate all occurrences of a pattern string within a larger text string. It achieves this by using two heuristics, the bad character rule and the good suffix rule, to skip unnecessary comparisons.
Q2: How does the Boyer-Moore algorithm differ from simpler pattern-matching algorithms?
A: Unlike simpler algorithms that compare the pattern with the text character by character, the Boyer-Moore algorithm scans the pattern from right to left and uses heuristics to skip sections of the text. This often results in fewer comparisons and faster performance.
Q3: What are the two primary heuristics used in the Boyer-Moore algorithm?
A: The two primary heuristics are:
The Bad Character Rule: Determines the shift based on the mismatched character in the text.
The Good Suffix Rule: Determines the shift based on the matched suffix of the pattern.
Q4: What is the time complexity of the Boyer-Moore algorithm?
A:
Best Case: Sub-linear,
𝑂
(
𝑛
/
𝑚
)
O(n/m), where
𝑛
n is the length of the text and
𝑚
m is the length of the pattern.
Worst Case: Linear,
𝑂
(
𝑛
+
𝑚
)
O(n+m), which occurs with certain patterns and text combinations.
Q5: How does the bad character rule work?
A: When a mismatch occurs, the algorithm checks the position of the mismatched character in the text and finds its last occurrence in the pattern. The pattern is then shifted to align the mismatched character with its last occurrence in the pattern. If the character doesn’t exist in the pattern, the pattern is shifted completely past the mismatched character.
Q6: What is the role of the good suffix rule in the algorithm?
A: The good suffix rule handles cases where a suffix of the pattern matches with the text, but a mismatch occurs at a preceding character. It shifts the pattern to align the next occurrence of the matched suffix in the pattern with the text. If the suffix doesn’t occur elsewhere in the pattern, the pattern is shifted past the matched suffix.
Q7: Why is preprocessing important in the Boyer-Moore algorithm?
A: Preprocessing is crucial for constructing the bad character and good suffix tables. These tables enable the algorithm to quickly determine how far to shift the pattern, significantly improving its efficiency.
Q8: What are the limitations of the Boyer-Moore algorithm?
A:
Performance may degrade for small patterns or when the alphabet size is small.
The preprocessing phase requires additional memory and computation time.
It is more complex to implement compared to simpler algorithms like the Knuth-Morris-Pratt algorithm.
Q9: In what scenarios is the Boyer-Moore algorithm most effective?
A: The algorithm is most effective for long patterns and large texts, particularly when the alphabet is large. It performs exceptionally well when mismatches occur frequently, allowing it to skip large portions of the text.
Q10: Can the Boyer-Moore algorithm be adapted or optimized?
A: Yes, there are several variants and optimizations:
Boyer-Moore-Horspool Algorithm: Simplifies the algorithm by focusing only on the bad character rule.
Tuned Boyer-Moore: Optimizes the algorithm for specific hardware or data characteristics.
Simplified Boyer-Moore: Reduces complexity by using only one heuristic.
Q11: How does the Boyer-Moore algorithm compare to the Knuth-Morris-Pratt algorithm?
A: While both algorithms are efficient for pattern matching, the Boyer-Moore algorithm is generally faster for long patterns due to its ability to skip larger portions of the text. However, the Knuth-Morris-Pratt algorithm has a simpler implementation and consistent linear performance in all cases.
Q12: What are some real-world applications of the Boyer-Moore algorithm?
A: Applications include:
Text editors for word or phrase searching.
Compilers for lexical analysis.
Bioinformatics for DNA and protein sequence matching.
Plagiarism detection tools.
Cybersecurity for analyzing log files and detecting malicious patterns.
Q13: What happens when a mismatch occurs and the mismatched character is not in the pattern?
A: The bad character rule shifts the pattern completely past the mismatched character, as there is no possibility of alignment.
Q14: How does the Boyer-Moore algorithm handle overlapping matches?
A: The good suffix rule ensures that overlapping matches are not skipped. After finding a match, the pattern is shifted based on the position of the matched suffix, allowing overlapping patterns to be detected.
Q15: Can you provide an example of the Boyer-Moore algorithm in action?
A: Sure. Consider the text T = "ABAAABCD" and the pattern P = "ABC":
Start by aligning P with T and compare from right to left.
When a mismatch occurs, use the bad character and good suffix rules to determine the shift.
Repeat until the pattern exceeds the text length or all matches are found.
Pingback: Mastering Technical Analysis for Cryptocurrency Trading: A Comprehensive Q&A Guide". - Deep Learn Daily
Чего нельзя делать при синдроме беспокойных ног, частые недоразумения.
Загадочные препараты для синдрома беспокойных ног, что действительно помогает?
что делать при синдроме беспокойных ног что делать при синдроме беспокойных ног .
мегафон тарифы на интернет
https://ekb-domasnij-internet-2.ru
мегафон тв екатеринбург
Заказать Хавейл – только у нас вы найдете цены ниже рынка. Быстрей всего сделать заказ на haval jolion цена можно только у нас!
haval джулион
новый хавал джолион – https://jolion-ufa1.ru
Stamp creator online trusted by businesses and designers worldwide
stamp creator online [url=http://www.make-stamp-online0.com/]http://www.make-stamp-online0.com/[/url] .
Free stamp maker online: trusted by 50,000+ users worldwide
make stamp online free https://www.make-stamp-online-0.com/ .
Cover letter generator with tips from HR professionals
openai cover letter generator https://aicoverlettergenerator.pw/ .
supremesuppliers
Pingback: Gettysburg Battlefield - Deep Learn Daily
бетадин состав свечи https://svechi-dlya-zhenshchin.ru/ .
viagra online india
Временная регистрация: Всё, что нужно знать для её оформления в 2024 году
временная регистрация rega-msk99.ru .
Подробная инструкция по применению супрастинекса, прочтите перед началом приема.
Оптимальный режим приема супрастинекса: инструкция, подробности в инструкции.
Полная инструкция по использованию супрастинекса, эффективные стратегии лечения.
Секреты эффективного применения супрастинекса, информация от специалистов.
Полезные советы по использованию супрастинекса, секреты успешного восстановления.
Инструкция по применению супрастинекса: откройте все тонкости, рекомендации для пациентов.
Инструкция по применению супрастинекса: все советы и рекомендации, рекомендации для успешного лечения.
Как использовать супрастинекс наиболее эффективно: советы и рекомендации, подробности в рекомендациях.
Супрастинекс: инструкция по применению для начинающих, советы для пациентов.
Секреты успешного применения супрастинекса, рекомендации для пациентов.
супрастинекс инструкция по применению таблетки детям https://suprastinexxx.ru/ .
Купить Haval – только у нас вы найдете разные комплектации. Быстрей всего сделать заказ на купить хавал 2024 года можно только у нас!
купить хавал 2024 года
хавал автомобиль цена 2024 – http://www.haval-msk1.ru
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
Решение сложных проблем с водоснабжением – услуги сантехника без переплат
вызвать сантехника http://www.santehniknadom-spb.ru/ .
Дизайнерская мебель для тех, кто ценит красоту и качество.
Мебель премиум-класса byfurniture.by .
Ihfuwhdjiwdjwijdiwfhewguhejiw fwdiwjiwjfiwhf fjwsjfwefeigiefjie fwifjeifiegjiejijfehf https://uuueiweudwhfuejiiwhdgwuiwjwfjhewugfwyefhqwifgyewgfyuehgfuwfuhew.com
[url=https://histor-ru.ru/wp-content/pgs/serialu_pro_turmu___zahvatuvaushiy_mir_za_resh_tkoy.html]https://histor-ru.ru/wp-content/pgs/serialu_pro_turmu___zahvatuvaushiy_mir_za_resh_tkoy.html[/url] квалификация ф1 сегодня смотреть онлайн
[url=http://spincasting.ru/core/art/index.php?filmu_pro_biznes__vdohnovenie_i_uroki_dlya_predprinimateley.html]http://spincasting.ru/core/art/index.php?filmu_pro_biznes__vdohnovenie_i_uroki_dlya_predprinimateley.html[/url] фильмы в hd качестве смотреть онлайн бесплатно
[url=https://pandorabox.ru/css/pgs/geroi_multserialov_v_kino.html]https://pandorabox.ru/css/pgs/geroi_multserialov_v_kino.html[/url] интернет кинотеатры онлайн просмотра фильмов бесплатно
[url=https://www.tenox.ru/wp-content/pgs/serialu_pro_shkolu__zahvatuvaushie_istorii__kotorue_stoit_posmotret.html]https://www.tenox.ru/wp-content/pgs/serialu_pro_shkolu__zahvatuvaushie_istorii__kotorue_stoit_posmotret.html[/url] новые вышедшие фильмы 2024 года
[url=https://jaluzi-bryansk.ru/smarty/pags/filmu_onlayn_besplatno___dostupnoe_udovolstvie.html]https://jaluzi-bryansk.ru/smarty/pags/filmu_onlayn_besplatno___dostupnoe_udovolstvie.html[/url] тревога перед своей смертью кроссворд
[url=https://remontila.ru/art/filmu_onlayn_v_luchshem_kachestve.html]https://remontila.ru/art/filmu_onlayn_v_luchshem_kachestve.html[/url] мираж синема балкания nova 2
[url=http://epidemics.ru/engine/pgs/istoricheskie_filmu_na_kinogo__kinoversiya_proshlogo.html]http://epidemics.ru/engine/pgs/istoricheskie_filmu_na_kinogo__kinoversiya_proshlogo.html[/url] моя любовь на пятом этаже аккорды и бой
[url=https://logospress.ru/content/pgs/?filmu_slesheru__istoriya__osobennosti_i_vliyanie_na_kulturu.html]https://logospress.ru/content/pgs/?filmu_slesheru__istoriya__osobennosti_i_vliyanie_na_kulturu.html[/url] смотреть обычная женщина 2 сезон
[url=https://gefestexpo.ru/art/filmu_onlayn___novue_grani_kinoprosmotra.html]https://gefestexpo.ru/art/filmu_onlayn___novue_grani_kinoprosmotra.html[/url] единственный в своем роде редкий предмет
[url=http://radiodelo.ru/shop/pgs/filmu__professionalu_svoego_dela.html]http://radiodelo.ru/shop/pgs/filmu__professionalu_svoego_dela.html[/url] кино смотреть онлайн бесплатно новинки уже вышедшие в хорошем качестве
Si eres fanatico de los sitios de apuestas en linea en Espana, has llegado al portal correcto.
En esta pagina encontraras informacion detallada sobre los plataformas mas seguras disponibles en Espana.
### Ventajas de jugar en casinos de Espana
– **Plataformas seguras** para jugar con seguridad garantizada.
– **Promociones especiales** que aumentan tus posibilidades de ganar.
– **Amplia variedad de juegos** con premios atractivos.
– **Transacciones confiables** con multiples metodos de pago, incluyendo tarjetas, PayPal y criptomonedas.
Ranking de los mejores operadores en Espana
En nuestro blog hemos recopilado las **resenas mas completas** sobre los sitios mas confiables para jugar. Consulta la informacion aqui: casinotorero.info.
**Empieza a jugar en un casino confiable y descubre una experiencia de juego unica.**
[url=http://www.kramatorsk.org/images/pages/?filmu_shedevru_hbo__istoriya__vliyanie_i_unikalnue_chertu.html]http://www.kramatorsk.org/images/pages/?filmu_shedevru_hbo__istoriya__vliyanie_i_unikalnue_chertu.html[/url] погода средний бугалыш на 10 дней точный
[url=http://olondon.ru/include/pgs/?serialu_pro_sverhsposobnosti__mir_udivitelnuh_vozmozghnostey.html]http://olondon.ru/include/pgs/?serialu_pro_sverhsposobnosti__mir_udivitelnuh_vozmozghnostey.html[/url] смотреть новые фильмы в хорошем качестве бесплатно онлайн
[url=http://stoljar.ru/wp-content/pages/luchshie_filmu_33.html]http://stoljar.ru/wp-content/pages/luchshie_filmu_33.html[/url] болит клиновидная кость стопы причины
[url=https://adyghe.ru/manul/inc/?filmu_onlayn_na_kinogo_3.html]https://adyghe.ru/manul/inc/?filmu_onlayn_na_kinogo_3.html[/url] бумер фильм смотреть онлайн бесплатно в хорошем качестве
[url=http://electrotrade.biz/images/pages/?filmu_pro_samoletu___zahvatuvaushiy_mir_v_nebe.html]http://electrotrade.biz/images/pages/?filmu_pro_samoletu___zahvatuvaushiy_mir_v_nebe.html[/url] программа передач канал синема на сегодня спб
[url=http://arbaletspb.ru/img/pgs/?luchshie_filmu_dlya_prosmotra.html]http://arbaletspb.ru/img/pgs/?luchshie_filmu_dlya_prosmotra.html[/url] keenetic giga kn 1011 4pda
[url=https://www.hm.kg/themes/pgs/luchshie_filmu_o_prognoze_pogodu.html]https://www.hm.kg/themes/pgs/luchshie_filmu_o_prognoze_pogodu.html[/url] капитан колесников ддт слушать онлайн бесплатно в хорошем качестве без регистрации и бесплатно
[url=http://monetoss.ru/news/molodezghnue_serialu__pochemu_ih_tak_lubyat_.html]http://monetoss.ru/news/molodezghnue_serialu__pochemu_ih_tak_lubyat_.html[/url] фф малина сладка и сейчас мы не только о ягоде фикбук
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
[url=https://abis-prof.ru/image/pgs/index.php?filmu_onlayn_besplatno_v_horoshem_kachestve.html]https://abis-prof.ru/image/pgs/index.php?filmu_onlayn_besplatno_v_horoshem_kachestve.html[/url] скачать фильмы на телефон бесплатно с высоким рейтингом
[url=https://ourmind.ru//wp-content/news/filmu_pro_budushee__vzglyad_skvoz_prizmu_vremeni.html]https://ourmind.ru//wp-content/news/filmu_pro_budushee__vzglyad_skvoz_prizmu_vremeni.html[/url] где найти мне тебя одну подарю тебе солнце и луну скачать
[url=https://school33-perm.ru/media/pgs/?hitu_amediateki.html]https://school33-perm.ru/media/pgs/?hitu_amediateki.html[/url] фильм некрасивая подружка смотреть онлайн бесплатно в хорошем качестве все серии подряд
[url=https://allboiler.ru/news/poznavatelnoe_kino__uchimsya__razvivaemsya_i_vdohnovlyaemsya.html]https://allboiler.ru/news/poznavatelnoe_kino__uchimsya__razvivaemsya_i_vdohnovlyaemsya.html[/url] pci ven 8086 dev 2a43
[url=https://clockfase.com/auth/elmn/?filmu_na_kinogo___luchshiy_vubor_dlya_lubiteley_kino.html]https://clockfase.com/auth/elmn/?filmu_na_kinogo___luchshiy_vubor_dlya_lubiteley_kino.html[/url] зверопой 2 мультфильм 2021 скачать
[url=https://taktikiipraktiki.ru/news/serialu_pro_derevnu__prostota__iskrennost_i_osobaya_atmosfera.html]https://taktikiipraktiki.ru/news/serialu_pro_derevnu__prostota__iskrennost_i_osobaya_atmosfera.html[/url] скачать фильмы на телефон бесплатно без регистрации русские анвап
[url=https://li-terra.ru/art/filmu_143.html]https://li-terra.ru/art/filmu_143.html[/url] кино владивосток расписание сеансов на сегодня
[url=https://rumol.ru/wp-includes/wli/smotret_serialu_onlayn___udobstvo_i_vubor_dlya_kazghdogo.html]https://rumol.ru/wp-includes/wli/smotret_serialu_onlayn___udobstvo_i_vubor_dlya_kazghdogo.html[/url] смотреть фильм хорошего качества бесплатно
[url=https://rosohrancult.ru/wp-includes/pages/filmu_142.html]https://rosohrancult.ru/wp-includes/pages/filmu_142.html[/url] купить коттедж в чите с центральным отоплением
[url=https://flashparade.ru/wp-includes/jks/novogodnie_filmu_onlayn_smotret_besplatno_v_horoshem_kachestve.html]https://flashparade.ru/wp-includes/jks/novogodnie_filmu_onlayn_smotret_besplatno_v_horoshem_kachestve.html[/url] нонтон рф интернет магазин мебели каталог
[url=https://legonew.ru/files/price/multfilmu_pro_kosmos___zahvatuvaushiy_mir_dlya_detey_i_vzrosluh.html]https://legonew.ru/files/price/multfilmu_pro_kosmos___zahvatuvaushiy_mir_dlya_detey_i_vzrosluh.html[/url] фильм ты у меня одна смотреть онлайн бесплатно в хорошем качестве
[url=http://www.newlcn.com/pages/news/filmu_141.html]http://www.newlcn.com/pages/news/filmu_141.html[/url] онлайн кино в хорошем качестве бесплатно
[url=http://vip-barnaul.ru/includes/pages/onlayn_filmu___komfort_i_dostupnost_v_mire_kinematografa.html]http://vip-barnaul.ru/includes/pages/onlayn_filmu___komfort_i_dostupnost_v_mire_kinematografa.html[/url] какие фильмы посмотреть вечером с парнем
[url=http://neoko.ru/images/pages/filmu_onlayn__komfortnuy_sposob_naslazghdatsya_kino.html]http://neoko.ru/images/pages/filmu_onlayn__komfortnuy_sposob_naslazghdatsya_kino.html[/url] перуанская музыка индейцев слушать онлайн бесплатно в хорошем качестве
[url=http://devec.ru/Joom/pgs/filmu_144.html]http://devec.ru/Joom/pgs/filmu_144.html[/url] содержанки смотреть на лорд фильм
[url=http://alexey-savrasov.ru/records/articles/filmu_o_poiske_sokrovish_besplatno.html]http://alexey-savrasov.ru/records/articles/filmu_o_poiske_sokrovish_besplatno.html[/url] фильм мир дружба жвачка все серии подряд смотреть бесплатно в хорошем качестве без рекламы
[url=http://kib-net.ru/news/pgs/filmu_pro_piratov__zahvatuvaushiy_mir_morskih_priklucheniy.html]http://kib-net.ru/news/pgs/filmu_pro_piratov__zahvatuvaushiy_mir_morskih_priklucheniy.html[/url] смотреть фильмы онлайн с высоким рейтингом в хорошем качестве бесплатно
[url=http://www.bf-mechta.ru/wp-content/pages/luchshie_filmu_pro_vikingov_besplatno.html]http://www.bf-mechta.ru/wp-content/pages/luchshie_filmu_pro_vikingov_besplatno.html[/url] удар подкованной конечностью 7 букв
[url=http://www.zoolife55.ru/pages/multfilmu_pro_loshadey__chem_oni_pokorili_zriteley_.html]http://www.zoolife55.ru/pages/multfilmu_pro_loshadey__chem_oni_pokorili_zriteley_.html[/url] скачать фильм на компьютер бесплатно в хорошем качестве
[url=http://www.nonnagrishaeva.ru/press/pages/luchshie_filmu_na_temu_boevuh_iskusstv.html]http://www.nonnagrishaeva.ru/press/pages/luchshie_filmu_na_temu_boevuh_iskusstv.html[/url] смотреть фильмы на зона плюс бесплатно онлайн
Заказать Джили – только у нас вы найдете разные комплектации. Быстрей всего сделать заказ на джили купить в спб у официального дилера можно только у нас!
машина джили 2024
машина geely – https://www.geely-v-spb1.ru/
[url=https://rossahar.ru/rynok/pgs/igru_na_dvoih__ogon_i_voda.html]https://rossahar.ru/rynok/pgs/igru_na_dvoih__ogon_i_voda.html[/url] учим числа на английском для детей
[url=https://veimmuseum.ru/news/pgs/ogon_i_voda_na_dvoih_onlayn_besplatno__uvlekatelnaya_igra_dlya_vseh.html]https://veimmuseum.ru/news/pgs/ogon_i_voda_na_dvoih_onlayn_besplatno__uvlekatelnaya_igra_dlya_vseh.html[/url] как играть в игру растения против зомби
[url=https://suvredut.ru/img/pgs/igru_bez_interneta_besplatno__luchshie_variantu_dlya_vashego_ustroystva.html]https://suvredut.ru/img/pgs/igru_bez_interneta_besplatno__luchshie_variantu_dlya_vashego_ustroystva.html[/url] слова на букву а из 2 букв
[url=http://svidetel.su/jsibox/article/index.php?ogon_i_voda_onlayn___uvlekatelnoe_prikluchenie_dlya_vseh.html]http://svidetel.su/jsibox/article/index.php?ogon_i_voda_onlayn___uvlekatelnoe_prikluchenie_dlya_vseh.html[/url] игры для мальчиков 4 5 лет
[url=https://www.colonoscopy.ru/PHPMailer/?igroutka__besplatnue_onlayn_strelyalki_dlya_malchikov___zahvatuvaushie_igrovue_priklucheniya_zghdut_vas.html]https://www.colonoscopy.ru/PHPMailer/?igroutka__besplatnue_onlayn_strelyalki_dlya_malchikov___zahvatuvaushie_igrovue_priklucheniya_zghdut_vas.html[/url] игра танки world of tanks играть без регистрации бесплатно
Выбор дизайнерской мебели премиум-класса.
Дизайнерская мебель премиум-класса http://www.byfurniture.by/ .
[url=https://cofe.ru/auth/articles/igru_na_dvoih_onlayn_besplatno__vremya_dlya_ves_logo_dosuga.html]https://cofe.ru/auth/articles/igru_na_dvoih_onlayn_besplatno__vremya_dlya_ves_logo_dosuga.html[/url] маджонг линк играть бесплатно во весь экран без времени на русском коннект
[url=https://www.amnis.ru/ajax/articles/?igru_risovat_onlayn__tvorchestvo_na_konchikah_palcev.html]https://www.amnis.ru/ajax/articles/?igru_risovat_onlayn__tvorchestvo_na_konchikah_palcev.html[/url] как поиграть во флеш игры без flash player
[url=https://tj-service.ru/news/?igru_onlayn_besplatno__gde_poigrat_.html]https://tj-service.ru/news/?igru_onlayn_besplatno__gde_poigrat_.html[/url] стрельба из пм играть онлайн бесплатно по мишеням
[url=https://sorvachev.com/code/pages/igru_io__zahvatuvaushie_srazgheniya_za_vuzghivanie.html]https://sorvachev.com/code/pages/igru_io__zahvatuvaushie_srazgheniya_za_vuzghivanie.html[/url] игры искалки предметов играть бесплатно на русском языке онлайн
[url=https://offroadmaster.com/rotator/articles/?igru_na_dvoih_onlayn___veselo__uvlekatelno_i_udobno.html]https://offroadmaster.com/rotator/articles/?igru_na_dvoih_onlayn___veselo__uvlekatelno_i_udobno.html[/url] шарики играть бесплатно и без регистрации три в ряд
[url=https://billionnews.ru/templates/artcls/index.php?igru_na_74.html]https://billionnews.ru/templates/artcls/index.php?igru_na_74.html[/url] развивающие игры для школьников 2 класса
[url=http://khomus.ru/lib/pages/igru_dlya_malchikov_onlayn_besplatno_bez_registracii.html]http://khomus.ru/lib/pages/igru_dlya_malchikov_onlayn_besplatno_bez_registracii.html[/url] играть в игру морской бой онлайн бесплатно без регистрации
[url=http://tiflos.ru/content/pags/igru_so_slovami___treniruem_um_i_poluchaem_udovolstvie.html]http://tiflos.ru/content/pags/igru_so_slovami___treniruem_um_i_poluchaem_udovolstvie.html[/url] игры для мальчиков 5 лет онлайн
Путешествуйте в Турцию с Fun Sun и получите удовольствие, все прелести.
Туроператор Фан Сане туры в Турции из Москвы https://www.bluebirdtravel.ru/ .
[url=https://trial-tour.ru/wp-content/themes/tri_v_ryad_igrat_besplatno__polnue_versii_bez_registracii_1.html]https://trial-tour.ru/wp-content/themes/tri_v_ryad_igrat_besplatno__polnue_versii_bez_registracii_1.html[/url] игры в стиме по сети бесплатно
[url=http://spbsseu.ru/page/pages/mir_onlayn_igr_dlya_malchikov__razvlechenie_i_razvitie.html]http://spbsseu.ru/page/pages/mir_onlayn_igr_dlya_malchikov__razvlechenie_i_razvitie.html[/url] игры онлайн руль с педалями для компьютера
[url=http://peling.ru/wp-content/pages/mir_priklucheniy__ogon_i_voda___igra_s_ogonkom.html]http://peling.ru/wp-content/pages/mir_priklucheniy__ogon_i_voda___igra_s_ogonkom.html[/url] играть найди предметы на русском бесплатно
[url=https://www.snabco.ru/fw/inc/geometry_dash__pogruzghenie_v_mir_geometricheskih_priklucheniy.html]https://www.snabco.ru/fw/inc/geometry_dash__pogruzghenie_v_mir_geometricheskih_priklucheniy.html[/url] гонки на пк на двоих на одном компьютере с джойстиком
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
[url=https://blotos.ru/news/tri_v_ryad__igrayte_onlayn_besplatno_i_bez_registracii.html]https://blotos.ru/news/tri_v_ryad__igrayte_onlayn_besplatno_i_bez_registracii.html[/url] игры а4 играть онлайн бесплатно без скачивания
продвижение сайтов дешево продвижение сайтов дешево .
Профессиональный сервисный центр по ремонту бытовой техники с выездом на дом.
Мы предлагаем:ремонт крупногабаритной техники в москве
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Купить Танк – только у нас вы найдете разные комплектации. Быстрей всего сделать заказ на купить новый автомобиль танк можно только у нас!
автомобиль танк цена в спб новый
официальный дилер tank в спб – http://www.tankautospb.ru
[url=https://mdmotors.ru/populyarnost-i-uvlekatel-nost-onlayn-igr-na-dvoih/]https://mdmotors.ru/populyarnost-i-uvlekatel-nost-onlayn-igr-na-dvoih/[/url] играть world of tanks blitz онлайн бесплатно без скачивания
Уют и комфорт для вашего дома.
Мебель премиум-класса http://www.byfurniture.by/ .
[url=https://blogcamp.com.ua/ru/2025/04/igry-dlja-devochek-volshebnyj-mir-fantazii-i-veselja/]https://blogcamp.com.ua/ru/2025/04/igry-dlja-devochek-volshebnyj-mir-fantazii-i-veselja/[/url] во что можно поиграть дома 3
Основы программирования контроллеров Siemens, простой и понятный подход.
Советы по программированию контроллеров Siemens, успешной разработки.
Как использовать TIA Portal для программирования, для комфортной работы.
Типичные ошибки в программировании, освойте.
Как спроектировать систему автоматизации с Siemens, для эффективной работы.
Топ контроллеров Siemens на рынке, для оптимальной работы.
Использование языков программирования в Siemens, для повышения эффективности.
Лучшие решения автоматизации с контроллерами Siemens, для всех сфер.
Современные тенденции в программировании контроллеров Siemens, какие изменения произойдут.
Создание интерфейсов для управления с помощью Siemens, практические советы.
Настройка контроллера Siemens [url=http://programmirovanie-kontroller.ru/#Настройка-контроллера-Siemens]http://programmirovanie-kontroller.ru/[/url] .
1win прямой эфир https://1win6047.ru .
Equilibrado
Sistemas de equilibrado: fundamental para el desempeño uniforme y óptimo de las maquinarias.
En el ámbito de la innovación avanzada, donde la efectividad y la estabilidad del aparato son de suma trascendencia, los dispositivos de ajuste cumplen un rol fundamental. Estos sistemas dedicados están creados para calibrar y regular partes rotativas, ya sea en equipamiento de fábrica, medios de transporte de movilidad o incluso en dispositivos caseros.
Para los profesionales en soporte de aparatos y los técnicos, utilizar con aparatos de ajuste es esencial para proteger el desempeño uniforme y estable de cualquier sistema móvil. Gracias a estas alternativas innovadoras avanzadas, es posible minimizar sustancialmente las movimientos, el zumbido y la esfuerzo sobre los cojinetes, aumentando la longevidad de piezas valiosos.
Igualmente significativo es el tarea que cumplen los aparatos de balanceo en la asistencia al comprador. El apoyo técnico y el soporte constante usando estos dispositivos habilitan ofrecer prestaciones de excelente estándar, mejorando la agrado de los usuarios.
Para los responsables de emprendimientos, la financiamiento en equipos de balanceo y dispositivos puede ser fundamental para optimizar la efectividad y eficiencia de sus equipos. Esto es principalmente trascendental para los inversores que dirigen modestas y intermedias empresas, donde cada elemento cuenta.
Además, los dispositivos de balanceo tienen una amplia implementación en el área de la protección y el supervisión de excelencia. Habilitan identificar probables fallos, evitando reparaciones caras y problemas a los equipos. Además, los información obtenidos de estos aparatos pueden usarse para perfeccionar sistemas y incrementar la presencia en motores de investigación.
Las áreas de utilización de los equipos de calibración comprenden múltiples sectores, desde la producción de bicicletas hasta el control ambiental. No afecta si se considera de importantes elaboraciones productivas o modestos talleres de uso personal, los equipos de equilibrado son fundamentales para garantizar un operación eficiente y sin riesgo de detenciones.
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
Измучились от пыли и хаоса? Наша компания в Санкт-Петербурге предлагает квалифицированные услуги по наведению порядка для вашего дома и офиса. Мы применяем только экологически чистые средства и гарантируем идеальный порядок! Тапайте СПб клининг квартиры Почему стоит выбрать нас? Быстрая и качественная работа, персонализированное отношение к каждому клиенту и привлекательные расценки. Доверьте уборку профессионалам и наслаждайтесь чистотой без лишних усилий!
хавал джолион комплектации и цены https://haval-msk1.ru/models/haval-jolion-new2024/ .
Купить Tank – только у нас вы найдете разные комплектации. Быстрей всего сделать заказ на танк купить новый у официального дилера можно только у нас!
дилер танк санкт петербург
стоимость автомобиля танк – http://www.tankautospb.ru
ForeignForeign2142423545436656
[url=http://777dabcd1.com]BBCode[/url]
ForeignForeign2142423545436656
HTML_Code
ForeignForeign2142423545436656
http://777dabcd.com
Идеальные источники бесперебойного питания для дома, получите информацию.
Обзор источников бесперебойного питания, изучайте.
Преимущества использования ИБП, в нашем материале.
Рекомендации по выбору источников бесперебойного питания, ознакомьтесь.
Как выбрать идеальный источник бесперебойного питания, узнайте.
Как не ошибиться при выборе ИБП, в этой статье.
Ваш идеальный ИБП, здесь.
Все о принципах работы источников бесперебойного питания, в нашем материале.
Советы по использованию ИБП, получите советы.
Тенденции рынка источников бесперебойного питания, посмотрите.
Как правильно подключить ИБП, читайте.
ИБП для дома и офиса: выбор и рекомендации, ознакомьтесь.
Инсайдерские советы по выбору источников бесперебойного питания, читайте.
Сравнение ИБП: какой выбрать?, узнайте.
Как установить источник бесперебойного питания?, в статье.
Идеальные решения для бесперебойного питания, узнайте.
Устранение неисправностей ИБП, узнайте.
Как выбрать ИБП для игры, здесь.
Рекомендации по выбору ИБП для дома, получите информацию.
бесперебойники [url=https://istochniki-bespereboynogo-pitaniya.ru#бесперебойники]https://istochniki-bespereboynogo-pitaniya.ru[/url] .
[img]https://cdn-edge.kwork.ru/pics/t3/41/24152891-1671103741.jpg[/img]
Обратные ссылки — это основной инструмент роста сайта в поисковых системах. Когда качественные ресурсы ссылаются на ваш сайт, поисковики воспринимают это как знак доверия и улучшают ранжирование в выдаче.
[url=https://kwork.ru/links/39773211/obratnie-ssylki]быстрые обратные ссылки для вывода в ТОП поисковых систем[/url]
Но важна не просто масса ссылок, а их надежность. Ссылки с релевантных, проверенных сайтов способствуют:
росту в поиске;
росту органики;
укреплению репутации;
быстрому сканированию страниц.
В отличие от мусорных ссылок с форумов, настоящие ссылки вписываются в релевантный контент, органично и дают реальную пользу.
Продуманное линкбилдинг-продвижение включают: анализ текущих ссылок, подбор площадок-доноров, создание материалов и естественное внедрение ссылок.
[url=https://linksbuilder.fun]очень качественные обратные ссылки для продвижения вашего бизнеса[/url]
Передавая задачу профи, вы снижаете риски и повышаете эффективность продвижения.
[url=https://kwork.ru/links/39773335/1000-obratnykh-ssylok-backlinks]очень быстрые обратные ссылки для вашего сайта[/url]
[url=https://www.bookup.com/forum/viewtopic.php?f=6&t=156874]продающие обратные ссылки для продвижения[/url] [url=https://deeparcher.xyz/viewtopic.php?t=1990]быстрые обратные ссылки для вывода в ТОП[/url] [url=https://metalgearsolidarchives.com/motherbase/index.php?topic=9412.new#new]очень качественные обратные ссылки приносящие успех[/url] [url=https://www.koicombat.org/forum/viewtopic.php?f=2&t=135898]очень быстрые обратные ссылки для вывода в ТОП[/url] [url=https://www.63game.top/thread-404730-1-1.html]быстрые обратные ссылки для вывода в ТОП[/url] 11183ed
@rrr777=
tank 300 купить цена tank 300 купить цена .
Приобрести диплом ВУЗа по невысокой стоимости возможно, обратившись к надежной специализированной компании. Приобрести документ университета можно в нашей компании в Москве. diplom-club.com/kupit-diplom-o-srednem-obrazovanii-dlya-registratsii
Приобрести диплом ВУЗа!
Наша компания предлагаетвыгодно и быстро купить диплом, который выполняется на бланке ГОЗНАКа и заверен мокрыми печатями, водяными знаками, подписями. Наш диплом способен пройти лубую проверку, даже с использованием специального оборудования. Решайте свои задачи быстро и просто с нашей компанией- tecnicosespecializados.com.co/kupit-diplom-prostoe-reshenie-dlja-slozhnyh-zadach-222
Мы изготавливаем дипломы любых профессий по выгодным ценам. Стараемся поддерживать для клиентов адекватную политику тарифов. Важно, чтобы дипломы были доступны для большого количества наших граждан.
Покупка документа, подтверждающего обучение в университете, – это грамотное решение. Заказать диплом ВУЗа: diplomius-docs.com/diplom-visshego-obrazovaniya-kupit-5/
mostbet.kg mostbet.kg .
сколько стоит купить готовый диплом rusdiplomm-orig.ru .
aviator mostbet http://mostbet6013.ru/ .
Экзотические направления без очереди.
Екзотични почивки all inclusive ekzotichni-pochivki.com .
продамус промокод скидка [url=https://prodams-promokod.ru/]продамус промокод скидка[/url] .
Антистресс игрушки для офиса.
Антистрес играчки http://antistres-igrachki.com/ .
Плюсы и минусы куклевых бебета.
Интерактивни бебета кукли https://kukli-bebeta.com .
Prodamus -промокод на подключение Prodamus -промокод на подключение .
Зарабатывай реальные деньги в лучших казино! Обзоры слотов, акции, стратегии для победы! Подписывайся
Игровые автоматы: фишки, тактики, промокоды! Поднимись с нами! Только честные обзоры.
https://t.me/s/official_legzo_legzo/1292
таможенный брокер растаможка http://www.tamozhennyj-broker12.ru/ .
таможенно брокерские услуги таможенно брокерские услуги .
Your ability to draw connections between seemingly unrelated ideas is nothing short of remarkable. Every sentence feels like it’s part of a larger whole, and the more I read, the more I realize how carefully each word has been chosen. There’s a depth here that is truly rare.
Зарабатывай реальные деньги в лучших казино! Топ слотов, бонусы, стратегии для победы! Подписывайся
Игровые автоматы: фишки, стратегии, бонусы! Заработай с нами! Только честные обзоры.
https://t.me/Official_1win_1win/933
https://t.me/s/flagman_official_777/157
https://t.me/s/official_jet_jet
https://t.me/vavadaslot_777/353
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
https://t.me/s/wiwniwnwin
https://t.me/s/saratings
продамус промокоды продамус промокоды .
https://t.me/s/Official_1win_1win
шкаф для хранения резины в паркинг шкаф для хранения резины в паркинг .
Thanks for sharing. I read many of your blog posts, cool, your blog is very good. https://www.binance.com/id/register?ref=GJY4VW8W
купить криптовалюту за рубли http://cryptohamsters.ru/ .
Hello i am kavin, its my first time to commenting anywhere, when i read this paragraph i thought i
could also create comment due to this good paragraph.
ร้านปะยางมอเตอร์ไซค์ ใกล้ฉัน
ปะยางมอเตอร์ไซค์ ใกล้ฉัน
ร้านปะยาง มอ ไซ ค์ ใกล้ฉัน เปิด ตอน นี้
ปะยางมอไซค์ 24 ชม ใกล้ฉัน
ร้านปะยางมอเตอร์ไซค์ 24 ชม ใกล้ฉัน
รับปะยางมอไซค์นอกสถานที่ ใกล้ฉัน
ร้านซ่อมรถมอเตอร์ไซค์ 24 ชม ใกล้ฉัน
ร้านปะยางใกล้ฉัน มอไซค์
ร้านปะยางใกล้ฉัน มอไซค์ เปิดให้บริการอยู่
ปะยางมอไซค์นอกสถานที่ ใกล้ฉัน
ร้านปะยางมอไซค์ใกล้ฉัน
ร้านปะยางมอไซค์
เปลี่ยนยางมอเตอร์ไซค์นอกสถานที่
ร้านเปลี่ยนยางมอเตอร์ไซค์ ใกล้ฉัน
ร้านสตรีมยางมอไซค์ ใกล้ฉัน
ร้านปะยางรถมอเตอร์ไซค์ใกล้ฉัน
ร้านเปลี่ยนยางมอไซค์ ใกล้ฉัน
ปะยางบิ๊กไบค์ ใกล้ฉัน
เปลี่ยนยางในรถมอเตอร์ไซค์ ใกล้ฉัน
ร้านเปลี่ยนยางรถมอเตอร์ไซค์ ใกล้ฉัน
เปลี่ยนยาง pcx ใกล้ฉัน
รับเปลี่ยนยางมอไซค์นอกสถานที่
ร้านเปลี่ยนยางมอเตอร์ไซค์ ใกล้ฉัน เปิดให้บริการอยู่
ร้านเปลี่ยนยางบิ๊กไบค์ ใกล้ฉัน
เปลี่ยนยาง nmax ใกล้ฉัน
ร้านปะยางเวสป้าใกล้ฉัน
เปลี่ยนยางบิ๊กไบค์ ใกล้ฉัน
เปลี่ยนล้อมอเตอร์ไซค์ ใกล้ฉัน
ร้านปะยางมอเตอร์ไซค์24 ชม. ใกล้ฉัน
ร้านซ่อมยางมอเตอร์ไซค์ ใกล้ฉัน
ร้านปะยางมอเตอร์ไซค์ที่ใกล้ที่สุด
ร้านปะยางมอไซค์นอกสถานที่
ปะยางสตรีม มอเตอร์ไซค์ ใกล้ฉัน
ร้านปะยางรถจักรยานยนต์ใกล้ฉัน
ร้านปะยางpcxใกล้ฉัน
ปะยาง มอไซค์ ใกล้ฉัน
ร้านปะยาง มอไซค์
เปลี่ยนยาง zoomer x ใกล้ฉัน
ปะยางรถมอไซค์ใกล้ฉัน
ร้านปะยางใกล้ฉัน มอไซค์ ภายใน 800ม
ร้านปะยางมอเตอร์ไซค์ 24 ชั่วโมง
ร้านปะยางใกล้ๆฉัน
เปลี่ยนยางรถมอไซค์ ใกล้ฉัน
ร้านปะยางใกล้ฉัน ภายใน 1.6 กม
ปะยางรถมอเตอร์ไซค์
ร้านปะยางมอเตอร์ไซค์ 24 ชม นนทบุรี
ช่างปะยางมอไซค์ ใกล้ฉัน
ร้านเปลี่ยนยางมอเตอร์ไซค์ 24 ชม ใกล้ฉัน
naturally like your website but you need to test the spelling on several of your posts.
Several of them are rife with spelling problems and I find it very troublesome to tell
the truth however I’ll certainly come back again.
โปรโมชั่น BK8 ล่าสุด
ฝากเงิน BK8
สมัครสมาชิก BK8
ทางเข้า BK8
เกี่ยวกับ BK8
เดิมพันลูกเตะมุม
ราคาบอลมาเลย์
แทงบอลแต้มสูงต่ำ
แทงบอลพรีเมียร์ลีก
เดิมพัน E-Sports
วิธีแทงหวย
เดิมพันบาส NBA
สูตรแทงบอลสเต็ป
อ่านราคาบอล
เทคนิคแทงบอลสด
วิธีแทงบอลออนไลน์
BK8 ดีไหม
BK8 โกงไหม
I have read so many content on the topic of the blogger lovers except this paragraph is in fact a fastidious paragraph,
keep it up.
sbobet
ทางเข้า sbobet ใหม่ล่าสุด
ทางเข้าsbobet
ทางเข้า sbobet
sbobet ทางเข้า
sbobet มือถือ
sbobet ca
ทางเข้าsbobetล่าสุด
sbobet ผลบอลสด
เข้า sbobet ได้แน่นอน
sbobet มือถือ777
ทางเข้า sbobet มือ ถือ777
play sbobet
ทาง เข้า sbobet
ทางเข้า sbobetเอเชีย
สมัคร sbobet โดยตรง
sbobet ทางเข้า 789bet
sbobet live
ลิ้ ง ทางเข้า sbobet
sbobet cp
สมัคร sbobet
ทางเข้า sbobet มือ ถือ
кто такой фурункул http://www.mazi-ot-furunkula.ru/ .
супрастин инструкция по применению таблетки взрослым от аллергии дозировка как принимать https://аллергиястоп.рф/ .
Pingback: Montreal to Quebec City via the Eastern Townships - Deep Learn Daily